The Javascript double question mark ??
is known as the nullish coalescing operator. It is a logical operator useful for checking whether a value is undefined
or null
, allowing us to provide a default value based on that check.
How does the nullish coalescing operator work? 🧁
Suppose we want to decide on a party treat based on whether a cupcake is available. If the cupcake is missing (i.e. it’s undefined
or null
), we’ll go with the brownie instead.
const partyTreat = cupcake ?? brownie;
JavaScriptThe operator ??
in this snippet returns the right-hand operand – brownie
– when the left one – cupcake
– is null
or undefined
.
For those of you who immediately jumped, shouting that this is the same as the logical OR operator ||
, hang in there, I’ll get to you soon.
So, to make this crystal clear, let’s see the possible scenarios:
- If the cupcake is
null
orundefined
, then ourpartyTreat
will be abrownie
. - If the cupcake is anything other than
null
orundefined
, then ourpartyTreat
will be thecupcake
.
I just hope they actually bring a real cupcake, not a truthy version of it… 🥶 (A cold programmer’s joke 🤣).
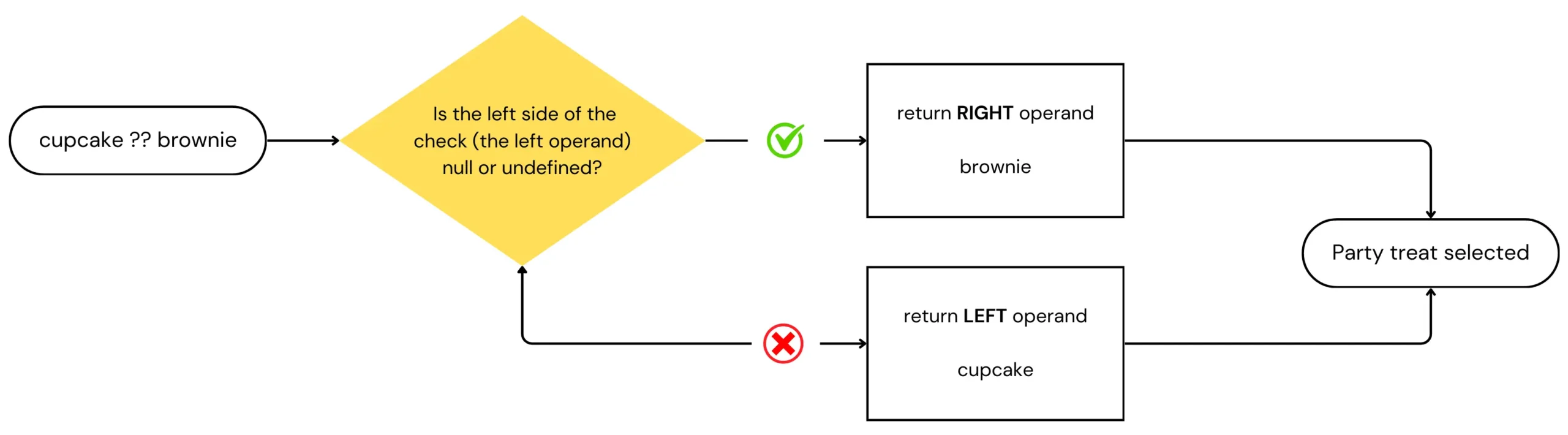
Choosing the Right Operator: ??
or ||
?
When it comes to handling default values in JavaScript, understanding the difference between the nullish coalescing operator (??
) and the logical OR operator (||
) is essential.
While both operators can provide fallback values, they behave differently with certain inputs.
The nullish coalescing operator returns the right-hand operand only if the left-hand operand is null
or undefined
, making it ideal for cases where you want to avoid falsy values like 0
or ''
.
In contrast, the logical OR operator returns the right-hand operand for any falsy value, including 0
, NaN
, or an empty string.
By now, I am sure you understand that the nullish coalescing operator is a special case of a logical ||
operator, right?
So, they are different, but what would be a use case where one operator is preferable over the other?
Let’s assume we have a function that gets us a user’s score
// Logical operator || example
function getUserScore() {
const finalScore = score || 100;
...
}
// Nullish coalescing operator ?? example
function getUserScore() {
const finalScore = score ?? 100;
...
}
JavaScriptIn the first case, if the score is 0
, which is falsy, then the finalScore
ends up being assigned the default value of 100
, even though 0
might be a legitimate score.
In the second case, that won’t happen. 0
is neither null
or undefined
, so the finalScore
will end being 0
, which is indeed a valid score.
Frequently Asked Questions 🤔
Can I combine the nullish coalescing operator with other operators in Javascript?
Yes, you can combine the nullish coalescing operator (??
) with other operators to create more complex logic in your code. For example, by writing something like
const defaultValue = hasUserInput ?? (isAdmin && fallbackValue);
JavaScriptwe first evaluate if the user has entered a value (such as in a form). If userInput
is null
or undefined
, we then evaluate whether the user is an admin.
If the user is an admin, result
will be assigned the fallbackValue
. If the user is not an admin, result
will be false
instead.
How can I chain multiple nullish coalescing operators together?
You can chain multiple nullish coalescing operators to evaluate different variables. For example, if you have several fallback options, the operator will return the first defined value it encounters:
const partyTreat = cupcake ?? brownie ?? defaultTreat;
JavaScriptHave you encountered any situations where one operator has significantly impacted your coding experience? If so, please share your examples in the comments below. We’d love to hear your insights!