Hello everybody! In this post, we will analyze the CSS first child selector, one of the most useful selectors in CSS. We utilize the CSS first-child
selector when we want to target and manipulate only the first element among a variety of elements of the same type. Note that when we refer to types of elements, we mean HTML tags like paragraphs (<p>
), headings (<h1>
, <h2>
…), lists (<li>
), and so on.
We often apply the CSS first child selector to list items (<li>
), but it can be used with any type of element. Below, I have prepared some examples to help you understand how to use the CSS first child selector depending on your needs.
Alternatively, we can use the CSS nth child selector like this :nth-child(1)
for the same purpose, but it’s more generic as it allows the selection of any child based on its position.
In addition to providing all the necessary CSS syntax for each first child case we describe, we have also included Sass syntax under each code snippet if you are using a Sass pre-processor.
CSS first child selector: List element
In the following example, the HTML structure consists of an ordered list (<ol>
) with three list items (<li>
), all colored indigo.
<ol>
<li>First item</li>
<li>Second item</li>
<li>Third item</li>
</ol>
HTML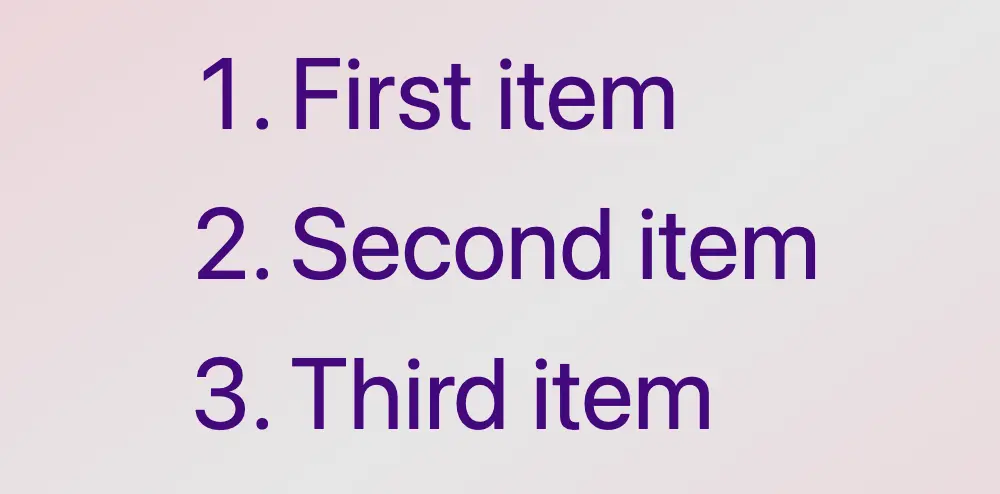
Then, we add the CSS rule, which selects only the first item and applies a red color to it.
/* Applies indigo color to all list items */
li {
color: indigo;
}
/* Applies red color only to the first list item */
li:first-child {
color: red;
}
/* OR */
li:nth-child(1) {
color: red;
}
CSS🔖 When we work with Sass, our code will be structured as follows:
li {
color: indigo;
&:first-child {
color: red;
}
}
/* OR */
li {
color: indigo;
&:nth-child(1) {
color: red;
}
}
SCSS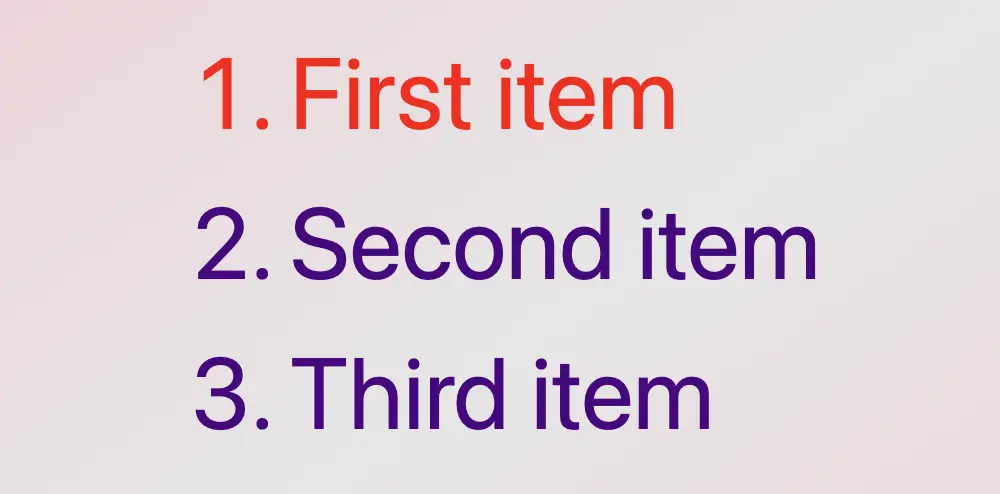
When using the :first-child
selector in CSS, it targets the very first element within its parent. When we add a new item to the beginning of the list, this new item becomes the first child of the list, and the previous first item now becomes the second one. This change causes the :first-child
selector to now target the newly added item instead.
CSS first child selector: Non-list element
In the example below, we set the first child selector for paragraphs. The HTML structure consists of a <div>
containing three paragraph (<p>)
elements. By default, all HTML elements have black text color, so paragraphs will appear in black.
<div>
<p>First paragraph</p>
<p>Second paragraph</p>
<p>Third paragraph</p>
</div>
HTML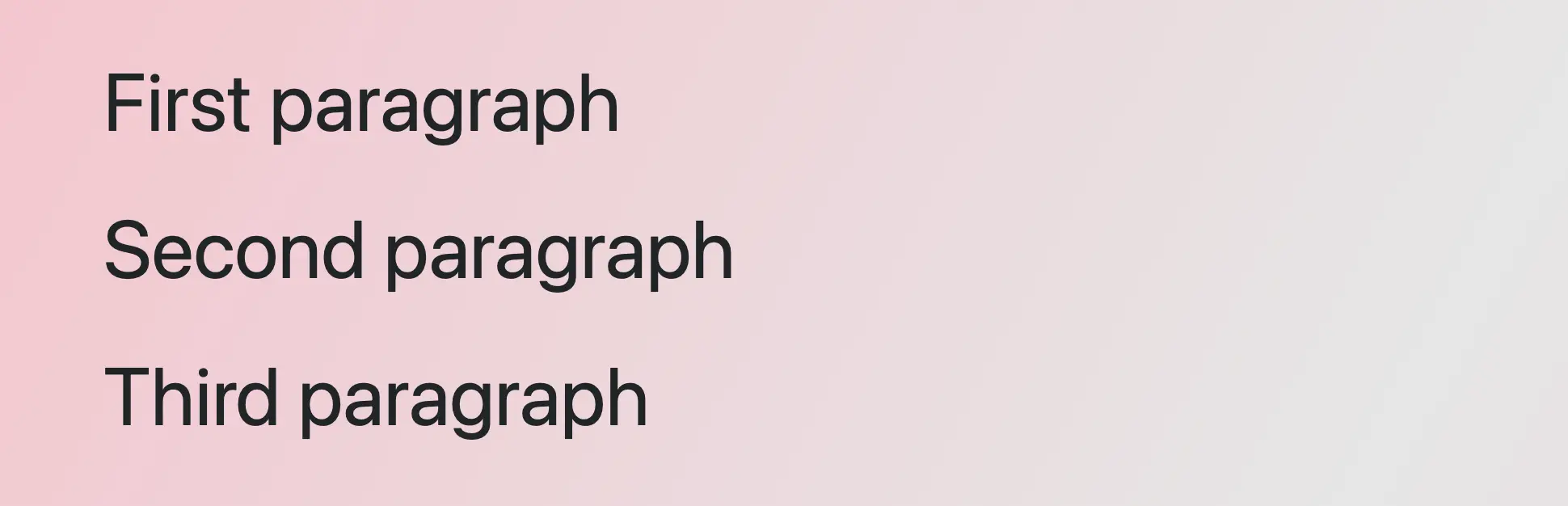
By applying the CSS first child selector, it selects the first <p>
element and apply the text color red to a white background. As a result, the first paragraph appears in red with a white background, while the subsequent paragraphs maintain their default styling.
/* Applies red color and background white
to the first paragraph
*/
p:first-child {
color: red;
background-color: white;
}
/* OR */
p:nth-child(1) {
color: red;
background: white;
}
CSS🔖 When working with Sass, our code will be structured as follows:
p {
&:first-child {
color: red;
background: white;
}
}
/* OR */
p {
&:nth-child(1) {
color: red;
background: white;
}
}
SCSS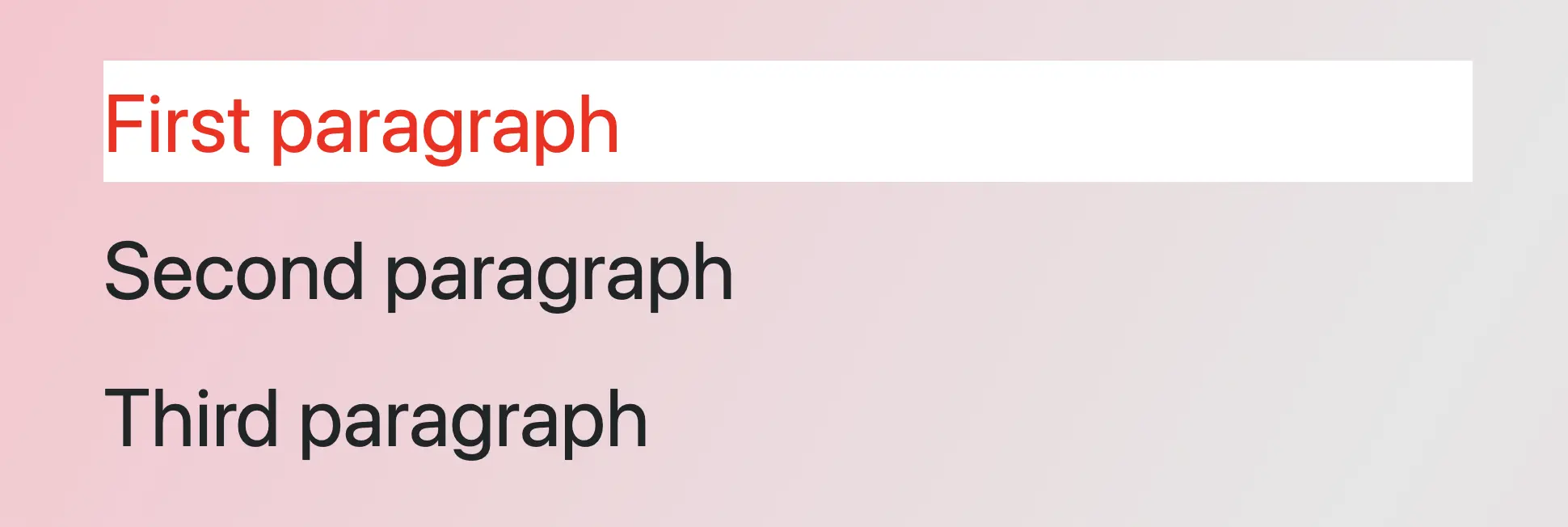
You may also like 🎬
First child CSS selector applied across multiple parent containers
In this example, we can see a different approach, as most times, we have multiple HTML elements to manipulate within a document. In our case, we have two div
elements, each containing two paragraph (<p>
) elements colored black.
<div>
<p>First paragraph inside the first div</p>
<p>Second paragraph inside the first div</p>
</div>
<div>
<p>First paragraph inside the second div</p>
<p>Second paragraph inside the second div</p>
</div>
HTML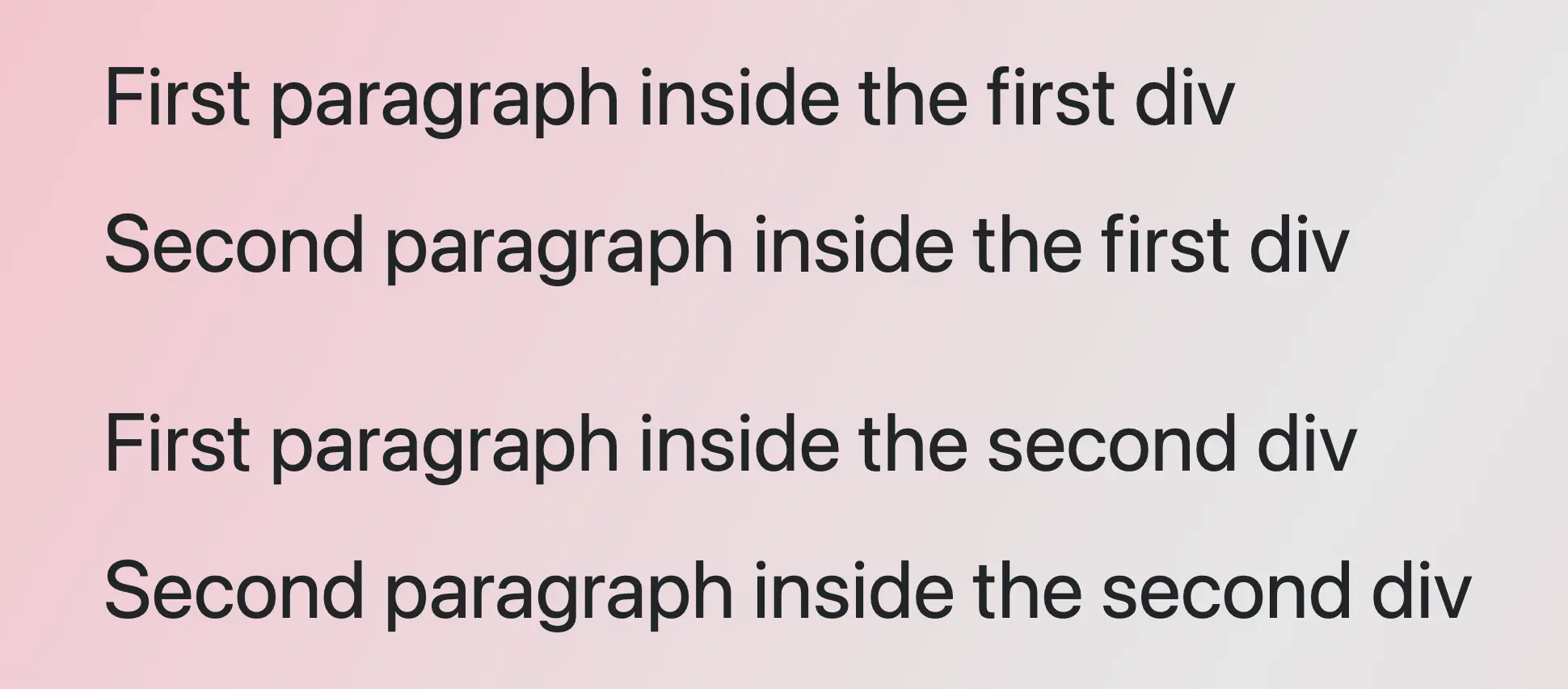
The CSS rule div p:first-child
selects the first <p>
element within any <div>
and applies the specified styles to it. As a result, the first paragraph within each <div>
will display in red with a white background, while the remaining paragraphs will retain their default styles. This demonstrates how the :first-child
selector can be used to style the first element within a number of parent containers. 😉
/* Applies red color and white background
to the first paragraph inside each div
*/
div p:first-child {
color: red;
background-color: white;
}
/* OR */
div p:nth-child(1) {
color: red;
background-color: white;
}
CSS🔖 When using Sass, our code will look like this:
div {
p:first-child {
color: red;
background-color: white;
}
}
/* OR */
div {
p:nth-child(1) {
color: red;
background-color: white;
}
}
SCSS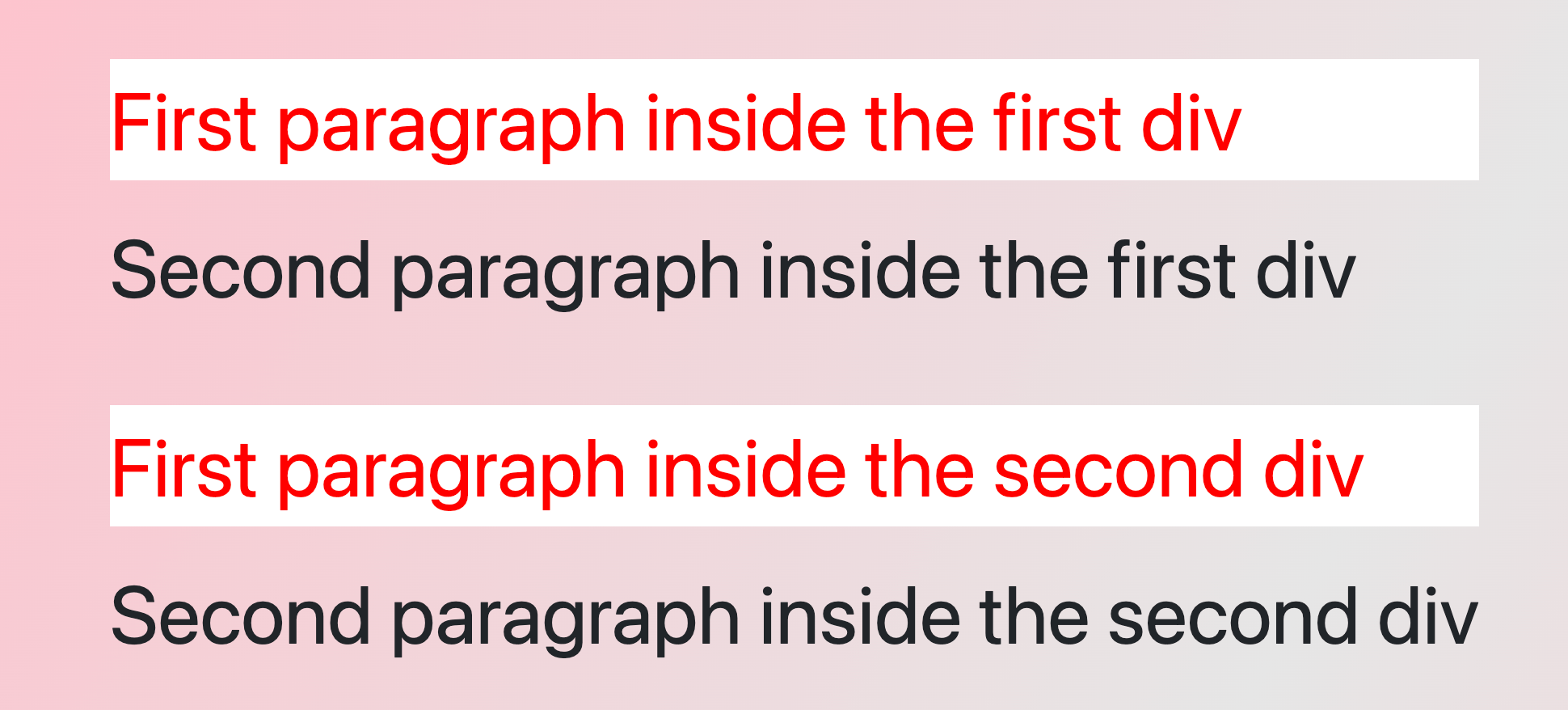
First child CSS selector targeting the first element of the first parent
In the following example, we have two div
elements, each containing two paragraph (<p>
) elements colored black.
<div>
<p>First paragraph in the first div</p>
<p>Second paragraph in the first div</p>
</div>
<div>
<p>First paragraph in the second div</p>
<p>Second paragraph in the second div</p>
</div>
HTML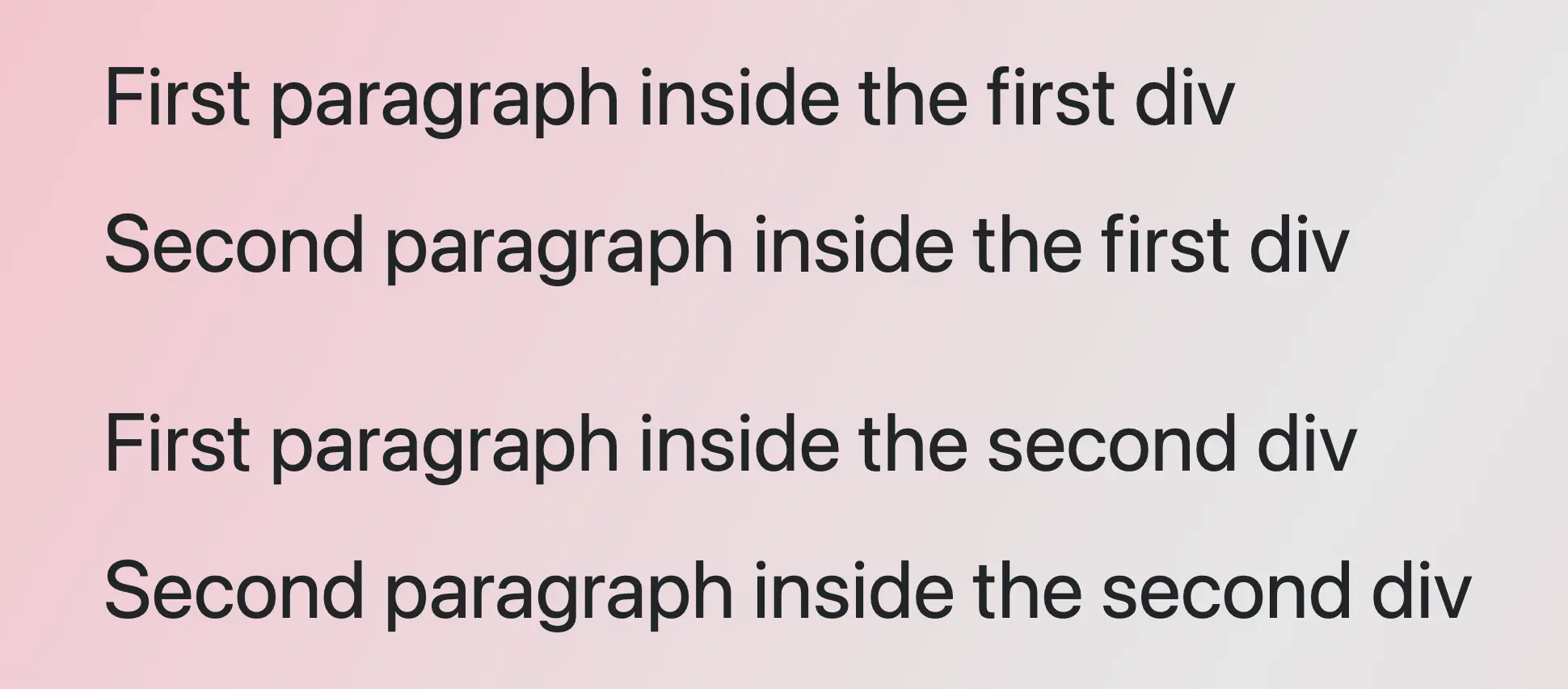
the CSS rule div:first-child p:first-child
selects only the first paragraph (<p>
) within the first div
and applies the specified styles to it. Thus, the first paragraph in the first div
will be displayed in red with a white background, while the second paragraph will remain in its default black color.
The second div
, remains unaffected by the styles applied to the first div
, showcasing how to apply specific styles selectively. 😉 This approach is really helpful for applying specific styles to elements in complex layouts.
/* Applies red color and white background
to the first paragraph of the first div
*/
div:first-child p:first-child {
color: red;
background-color: white;
}
/* OR */
div:nth-child(1) p:nth-child(1) {
color: red;
background-color: white;
}
CSS🔖 When we work with Sass, we will write our code as follows:
div:first-child {
p:first-child {
color: red;
background-color: white;
}
}
/* OR */
div:nth-child(1) {
p:nth-child(1) {
color: red;
background-color: white;
}
}
SCSS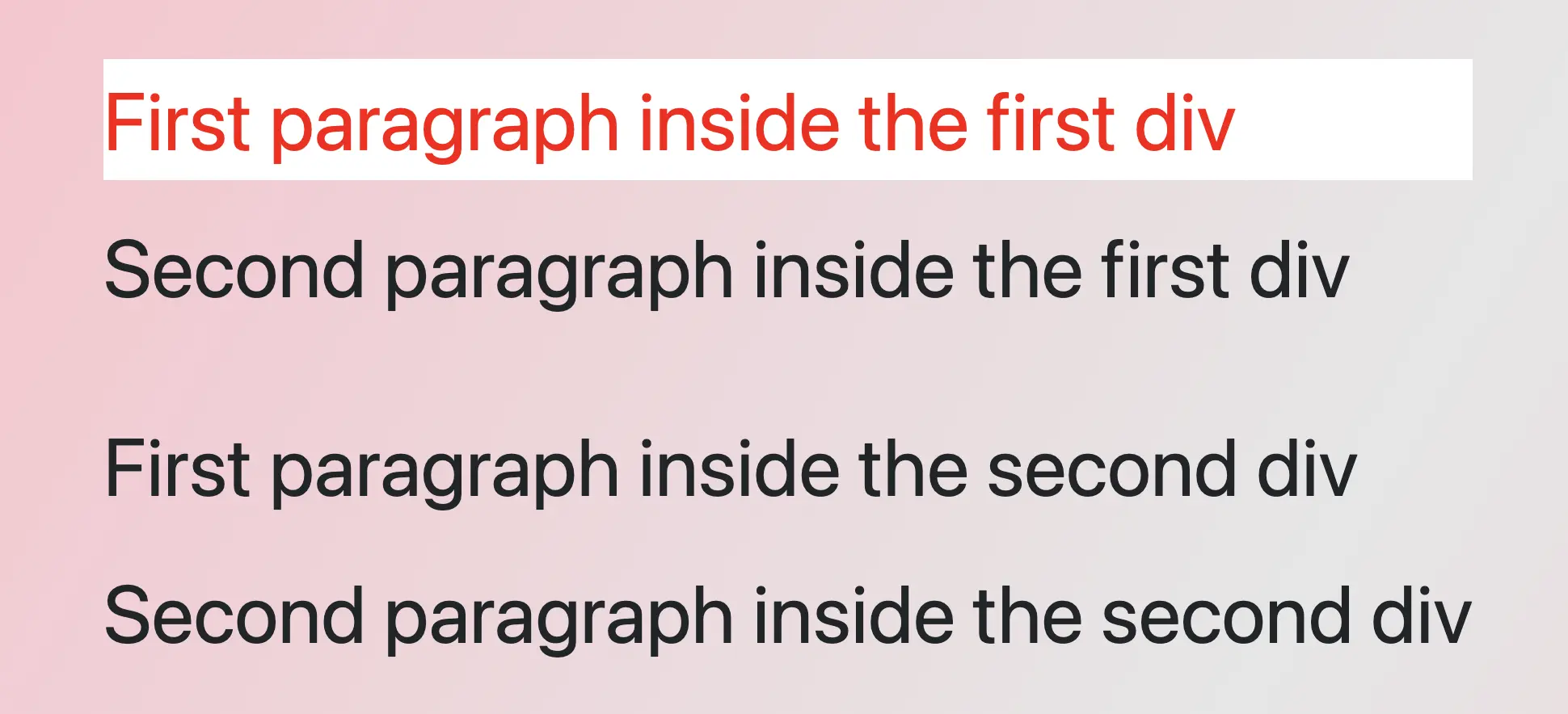
Using the :not(:first-child)
selector
In our last example, we have the opposite case. How not to select the CSS first child. The HTML structure consists of an ordered list <ol>
containing three list <li>
elements.
<ol>
<li>First item</li>
<li>Second item</li>
<li>Third item</li>
</ol>
HTML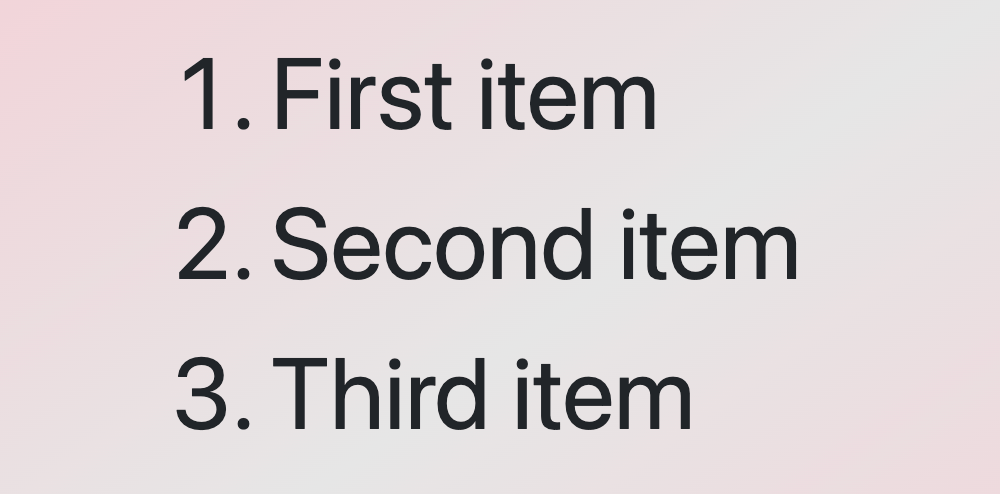
The CSS rule ol li:not(:first-child)
selects all <li>
elements within the ol
apart from the first one and applies the specified styles to them. Because of that, all list items apart from the first one will be displayed in red, while the first list item will retain its default color, black.
/* Applies red color to all paragraphs
except for the first one
*/
ol li:not(:first-child) {
color: red;
}
/* OR */
ol li:not(:nth-child(1)) {
color: red;
}
CSS🔖 When we work with Sass, we will write our code as follows:
ol {
li:not(:first-child) {
color: red;
}
}
/* OR */
ol {
li:not(:nth-child(1)) {
color: red;
}
}
SCSS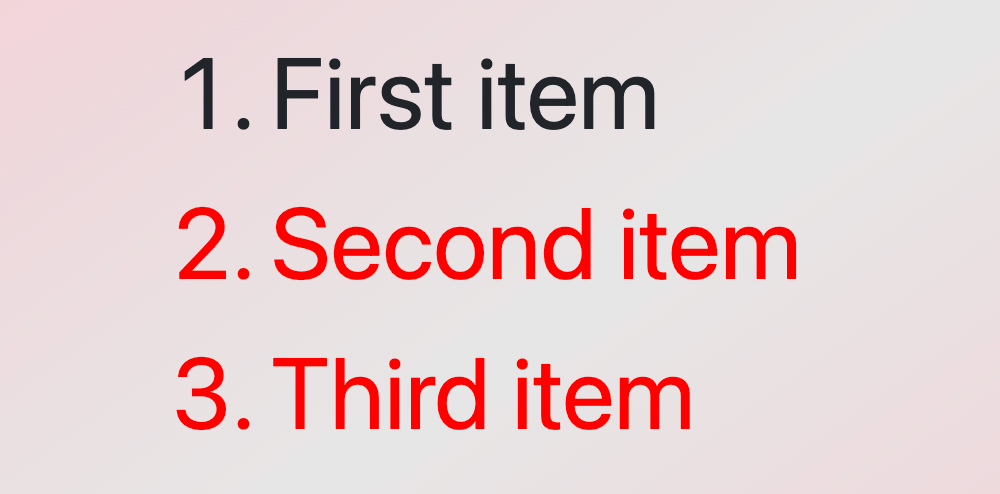
🌼 Hope you found my post interesting and helpful. Thanks for being here! 🌼